Given a 2D matrix matrix, find the sum of the elements inside the rectangle defined by its upper left corner (row1, col1) and lower right corner (row2, col2).
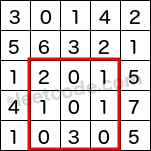
The above rectangle (with the red border) is defined by (row1, col1) = (2, 1) and (row2, col2) = (4, 3), which contains sum = 8.
Example:
Given matrix = [ [3, 0, 1, 4, 2], [5, 6, 3, 2, 1], [1, 2, 0, 1, 5], [4, 1, 0, 1, 7], [1, 0, 3, 0, 5] ] sumRegion(2, 1, 4, 3) -> 8 sumRegion(1, 1, 2, 2) -> 11 sumRegion(1, 2, 2, 4) -> 12
Note:
- You may assume that the matrix does not change.
- There are many calls to sumRegion function.
- You may assume that row1 ≤ row2 and col1 ≤ col2.
二维累计和,多种方法,binary indexed tree, segment tree都可以解。
不过此题要求是immutable, 不用更新,所以最简单的2d array是最优的
class NumMatrix { private int[][] rangeSum; public NumMatrix(int[][] matrix) { preProcess(matrix); } private void preProcess(int[][] matrix) { int m = matrix.length; if (m == 0) return; int n = matrix[0].length; rangeSum = new int[m][n]; for (int i = 0; i < m; i++) { int rowSum = 0; for (int j = 0; j < n; j++) { rowSum += matrix[i][j]; if (i == 0) { rangeSum[i][j] = rowSum; }else { rangeSum[i][j] = rowSum + rangeSum[i - 1][j]; } } } } public int sumRegion(int row1, int col1, int row2, int col2) { if (row1 == 0 && col1 == 0) { return rangeSum[row2][col2]; }else if (row1 == 0) { return rangeSum[row2][col2] - rangeSum[row2][col1 - 1]; }else if (col1 == 0) { return rangeSum[row2][col2] - rangeSum[row1 - 1][col2]; } return rangeSum[row2][col2] + rangeSum[row1 - 1][col1 - 1] - rangeSum[row2][col1 - 1] - rangeSum[row1 - 1][col2]; } } /** * Your NumMatrix object will be instantiated and called as such: * NumMatrix obj = new NumMatrix(matrix); * int param_1 = obj.sumRegion(row1,col1,row2,col2); */
303. Range Sum Query - Immutable
Given an integer array nums, find the sum of the elements between indices i and j (i ≤ j), inclusive.
Example:
Given nums = [-2, 0, 3, -5, 2, -1] sumRange(0, 2) -> 1 sumRange(2, 5) -> -1 sumRange(0, 5) -> -3
Note:
- You may assume that the array does not change.
- There are many calls to sumRange function.
class NumArray { private int[] rangeSum; public NumArray(int[] nums) { int n = nums.length; rangeSum = new int[n + 1]; for (int i = 0; i < nums.length; i++) { rangeSum[i + 1] += rangeSum[i] + nums[i]; } } public int sumRange(int i, int j) { return rangeSum[j + 1] - rangeSum[i]; } } /** * Your NumArray object will be instantiated and called as such: * NumArray obj = new NumArray(nums); * int param_1 = obj.sumRange(i,j); */
No comments:
Post a Comment