On an
N
xN
chessboard, a knight starts at the r
-th row and c
-th column and attempts to make exactly K
moves. The rows and columns are 0 indexed, so the top-left square is (0, 0)
, and the bottom-right square is (N-1, N-1)
.
A chess knight has 8 possible moves it can make, as illustrated below. Each move is two squares in a cardinal direction, then one square in an orthogonal direction.
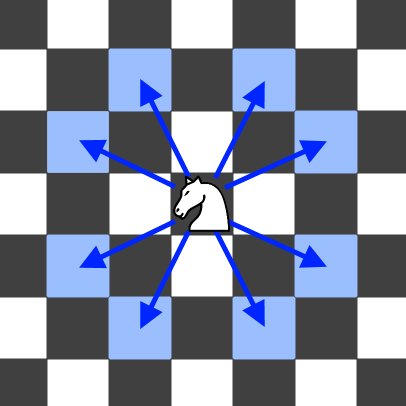
Each time the knight is to move, it chooses one of eight possible moves uniformly at random (even if the piece would go off the chessboard) and moves there.
The knight continues moving until it has made exactly
K
moves or has moved off the chessboard. Return the probability that the knight remains on the board after it has stopped moving.
Example:
Input: 3, 2, 0, 0 Output: 0.0625 Explanation: There are two moves (to (1,2), (2,1)) that will keep the knight on the board. From each of those positions, there are also two moves that will keep the knight on the board. The total probability the knight stays on the board is 0.0625.
Note:
N
will be between 1 and 25.K
will be between 0 and 100.典型的DP,按套路一路推理
Solution #1 超时
class Solution { public double knightProbability(int N, int K, int r, int c) { int total = 1; for (int i = 0; i < K; i++) { total *= 8; } return dfs(N, K, r, c) / total; } public double dfs(int N, int K, int r, int c) { if (r < 0 || r >= N || c < 0 || c >= N) { return 0; } if (K == 0) { return 1; } return dfs(N, K - 1, r - 2, c - 1) + + dfs(N, K - 1, r - 2, c + 1) + dfs(N, K - 1, r - 1, c - 2) + dfs(N, K - 1, r - 1, c + 2) + dfs(N, K - 1, r + 1, c - 2) + dfs(N, K - 1, r + 1, c + 2) + dfs(N, K - 1, r + 2, c - 1) + dfs(N, K - 1, r + 2, c + 1); } }
Solution #2 Memoization
O(N^2 * K) 时间
class Solution { private int[] dr = {-2, -2, -1, -1, 1, 1, 2, 2}; private int[] dc = {-1, 1, -2, 2, -2, 2, -1, 1}; public double knightProbability(int N, int K, int r, int c) { double[][][] dp = new double[N][N][K]; for (int i = 0; i < N; i++) { for (int j = 0; j < N; j++) Arrays.fill(dp[i][j], -1); } double a = dfs(N, K, r, c, dp); return a / Math.pow(8, K); } public double dfs(int N, int K, int r, int c, double[][][] dp) { if (r < 0 || r >= N || c < 0 || c >= N) { return 0; } if (K == 0) { return 1; } if (dp[r][c][K - 1] != -1) return dp[r][c][K - 1]; double total = 0; for (int i = 0; i < 8; i++) { total += dfs(N, K - 1, r + dr[i], c + dc[i], dp); } dp[r][c][K - 1] = total; return total; } }
Solution #3 DP, 思路同上。
因为[k]仅依赖于[k - 1], 所以用2个2维数组可以交替使用,不用3维
O(N^2 * K) 时间,O(N^2) 空间
class Solution { private int[] dr = {-2, -2, -1, -1, 1, 1, 2, 2}; private int[] dc = {-1, 1, -2, 2, -2, 2, -1, 1}; public double knightProbability(int N, int K, int r, int c) { double[][] dp1 = new double[N][N]; double[][] dp2 = new double[N][N]; dp1[r][c] = 1; for (int k = 0; k < K; k++) { for (int i = 0; i < N; i++) { for (int j = 0; j < N; j++) { if (dp1[i][j] > 0) { for (int d = 0; d < 8; d++) { helper(N, i, j, i + dr[d], j + dc[d], dp1, dp2); } dp1[i][j] = 0; } } } double[][] tmp = dp1; dp1 = dp2; dp2 = tmp; } double total = 0; for (int i = 0; i < N; i++) { for (int j = 0; j < N; j++) total += dp1[i][j]; } return total / Math.pow(8, K); } public void helper(int N, int r1, int c1, int r2, int c2, double[][] dp1, double[][] dp2) { if (r2 < 0 || r2 >= N || c2 < 0 || c2 >= N) { return ; } dp2[r2][c2] += dp1[r1][c1]; } }